Rust VM SDK: Build Custom Virtual Machines on Avalanche using Rust
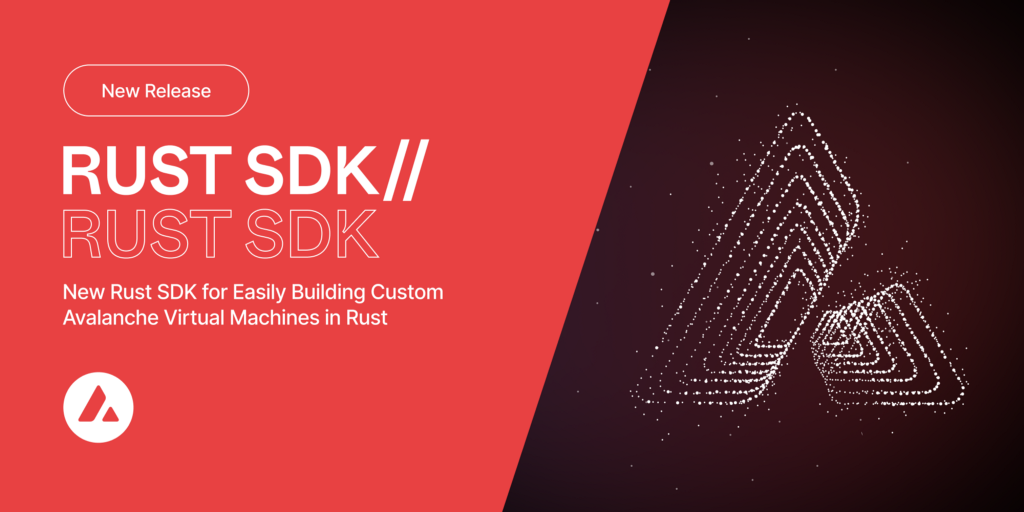
We’re excited to announce the release of our second SDK for building Avalanche Virtual Machines, the Rust SDK. As an alternative to using Golang, you can instead use Rust to launch your own blockchain on an Avalanche Subnet. Take a look at our TimestampVM example to get started (spoiler alert: the ONLY language you need to use when writing your Rust VM is Rust)!
At Ava Labs, we continue to invest in empowering creators by shipping SDKs and tools to make it as easy as possible to launch your own blockchain on top of Avalanche. We look forward to seeing what Rust devs build using this new SDK and are happy to provide support in Discord.
Primer: Building Your own Blockchain on Avalanche
To create your own blockchain on Avalanche, you need to first write your own Virtual Machine (VM). VMs define the logic that your blockchain will run and are capable of doing just about anything as long as it implements the Protocol Buffer (protobuf) interface exposed by AvalancheGo, which will communicate using this interface over gRPC.
The use of gRPC and protobuf enables consistent object models, thus improving the system’s understandability. The schema is defined in advance and can easily be extended with new information without invalidating the existing data. This communication model also enables developers to implement VMs in whatever language they want as long as it supports gRPC and protobuf. As mentioned previously, we currently have SDKs for Golang and Rust but plan to add others in the future.
More specifically, AvalancheGo is a client to a plugin server, and the virtual machine is the server that implements gRPC interfaces. Let’s take a look at an example in the `VM` service, initializing the VM:
message InitializeRequest {
uint32 network_id = 1;
bytes subnet_id = 2;
bytes chain_id = 3;
bytes node_id = 4;
bytes x_chain_id = 5;
bytes avax_asset_id = 6;
bytes genesis_bytes = 7;
bytes upgrade_bytes = 8;
bytes config_bytes = 9;
repeated VersionedDBServer db_servers = 10;
// server_addr is the address of the gRPC server which serves
// the messenger, keystore, shared memory, blockchain alias,
// subnet alias, and appSender services
string server_addr = 11;
}
The initialize request is made when AvalancheGo initializes the VM. Upon receipt of this request, the VM can set up the channel between the VM and the Snowman Consensus Engine, and configure the database instance managed by AvalancheGo, where the received genesis bytes can be stored.
Using the Rust SDK
Virtual machines in Golang use `hashicorp/go-plugin` as middleware to abstract the more menial aspects of plugin orchestration (logging, compatibility checks, etc.). Not only does the new Rust SDK implement this boilerplate orchestration logic, it also provides a set of libraries that simplifies VM and tool development in Rust. For example, `avalanche-ops` uses the Rust SDK to automate subnet installation on the cloud, and perform load tests using AWS KMS to sign transactions. The Rust SDK is published on crates.io.
Minimal VM implementations, such as `timestampvm-rs`, only require a few hundred lines of code and don’t require stitching in any other programming languages to build a fully-featured VM (even though the Avalanche node is written entirely in Golang). Here is an example of how to get started using `timestampvm-rs`:
[dependencies]
avalanche-types = { version = "0.0.x", features = ["subnet", "codec_base64"] }
use avalanche_types::subnet;
use timestampvm::vm;
use tokio::sync::broadcast::{self, Receiver, Sender};
#[tokio::main]
async fn main() -> std::io::Result<()> {
let (stop_ch_tx, stop_ch_rx): (Sender<()>, Receiver<()>) = broadcast::channel(1);
let vm_server = subnet::rpc::vm::server::Server::new(vm::Vm::new(), stop_ch_tx);
subnet::rpc::plugin::serve(vm_server, stop_ch_rx).await
}
Take a look at “How to Build a Simple Rust VM” for a full guide of how to build custom Virtual Machines using the new SDK.
Conclusion
We’re very excited about the potential Rust has for building efficient and scalable blockchains. We look forward to continuing to improve the SDK and working with the community on all sorts of novel blockchain designs. You can follow along by subscribing to the Rust SDK repository. If you have suggestions on how to improve the SDK, please open a PR!
About Avalanche
Avalanche is the fastest smart contracts platform in the blockchain industry, as measured by time-to-finality, and has the most validators securing its activity of any proof-of-stake protocol. Avalanche is blazingly fast, low cost, and green. Any smart contract-enabled application can outperform its competition by deploying on Avalanche. Don’t believe it? Try Avalanche today.
Website | Whitepapers | Twitter | Discord | GitHub | Documentation | Telegram | Facebook | LinkedIn | Reddit | YouTube
Rust VM SDK: Build Custom Virtual Machines on Avalanche using Rust was originally published in Avalanche on Medium, where people are continuing the conversation by highlighting and responding to this story.